Graphics Commands
This example program demonstrates some of the common graphics commands.
See the comments in the program for instructions on how to use each one. Here is
a copy: graphics_commands.cpp. (To save,
Right-click and do Save Target As...)
//
// Demonstration of Graphics functions
//
// Author: Matthew Weathers
// Date : September 8, 1999
#include <stdlib.h>
#include <math.h>
#include <time.h>
#include <string>
#include "graphics2.h"
#define ESC 0x1b
void main()
{
srand(time(NULL)); // Seed the random number generator
int GraphDriver=0,GraphMode=0;
initgraph( &GraphDriver, &GraphMode, "", 640, 480 ); // Start Window
//---------setcolor( color )
// Colors:
// BLACK, BLUE, GREEN, CYAN, RED, MAGENT, BROWN, LIGHTGRAY, DARKGRAY,
// LIGHTBLUE, LIGHTGREEN, LIGHTCYAN, LIGHTRED, LIGHTMAGENTA, YELLOW, WHITE
setcolor(WHITE);
//---------line( x1, y1, x2, y2);
line(20, 20, 100, 80);
setcolor(BLUE);
//---------rectangle( x1, y1, x2, y2);
rectangle(20, 100, 150, 150);
//---------setfillstyle( fill_style, color)
// Fill_styles:
// EMPTY_FILL, SOLID_FILL, LINE_FILL, LTSLASH_FILL, SLASH_FILL, BKSLASH_FILL,
// LTBKSLASH_FILL, HATCH_FILL, XHATCH_FILL, INTERLEAVE_FILL, WIDE_DOT_FILL,
// CLOSE_DOT_FILL, USER_FILL
setcolor(RED);
setfillstyle(SOLID_FILL, RED);
//---------bar( x1, y1, x2, y2);
bar(20, 200, 150, 220);
setfillstyle(SOLID_FILL, GREEN);
bar(20, 230, 150, 250);
setfillstyle(BKSLASH_FILL, LIGHTGREEN);
bar(160, 200, 190, 250);
//---------circle( X, Y, radius)
setcolor(WHITE);
circle( 50, 310, 40);
//---------putpixel (X, Y, color)
putpixel(50, 310, RED);
putpixel(60, 320, WHITE);
//---------arc( X, Y, start_angle, end_angle, radius);
// Angles in degrees 0-360.
// 0-right. Counter-clockwise (90-up, 180-left, 270-down)
arc( 140, 310, 0, 90, 40);
arc( 140, 310, 120, 160, 40);
//---------ellipse(x, y, start_angle, end_angle, x_radius, y_radius)
ellipse(60, 400, 0, 270, 50, 30);
//---------fillellipse(x, y, x_radius, y_radius);
setcolor(YELLOW);
setfillstyle(SOLID_FILL, YELLOW);
fillellipse(170, 400, 50, 30);
setcolor(WHITE);
setfillstyle(HATCH_FILL, RED);
fillellipse(250, 400, 30, 50);
//---------pieslice( x, y, start_angle, end_angle, radius)
setcolor(RED);
setfillstyle(SOLID_FILL, RED);
pieslice(350, 60, 0, 90, 40);
setcolor(WHITE);
setfillstyle(SOLID_FILL, BLUE);
pieslice(350, 60, 90,180, 40);
setcolor(GREEN);
setfillstyle(WIDE_DOT_FILL, WHITE);
pieslice(350, 65, 180, 270, 60);
setcolor(WHITE);
//---------setlinestyle(style, pattern, thickness);
// line styles:
// SOLID_LINE, DOTTED_LINE, CENTER_LINE, DASHED_LINE, USERBIT_LINE
// -- Normal: (SOLID_LINE, 0, 1);
setlinestyle(DOTTED_LINE, 0, 1);
line(300, 130, 500, 130);
setlinestyle(SOLID_LINE, 0, 3);
line(300, 150, 500, 150);
setlinestyle(DASHED_LINE, 0, 1);
rectangle(300, 170, 500, 200);
setlinestyle(SOLID_LINE, 0, 1); //Back to normal
line(300, 220, 500, 220);
//---------settextjustify( horizontal, vertical )
// horizontal = LEFT_TEXT, CENTER_TEXT, or RIGHT_TEXT
// vertical = BOTTOM_TEXT, TOP_TEXT
// -- Normal is (LEFT_TEXT, TOP_TEXT)
settextjustify(LEFT_TEXT,TOP_TEXT);
//---------settextstyle(font,direction,size)
// font = DEFAULT_FONT, TRIPLEX_FONT, SMALL_FONT, SANSSERIF_FONT, GOTHIC_FONT
// or try numbers 0 thru 15?
// direction = HORIZ_DIR, VERT_DIR
setcolor(YELLOW);
settextstyle(TRIPLEX_FONT, HORIZ_DIR, 2);
//---------outtextxy( x, y, "text")
outtextxy(300, 260, "Triplex, size 2");
setcolor(WHITE);
settextstyle(GOTHIC_FONT, VERT_DIR, 2);
outtextxy(600, 20, "Gothic, Vertical, Size 2");
setcolor(GREEN);
settextstyle(DEFAULT_FONT, HORIZ_DIR, 2);
outtextxy(300, 455, "Press any key...");
//---------getch()
getch(); //Wait for a key. (When main function ends, the window will close)
} // end of main()
Here's a screen shot of what the above program does:
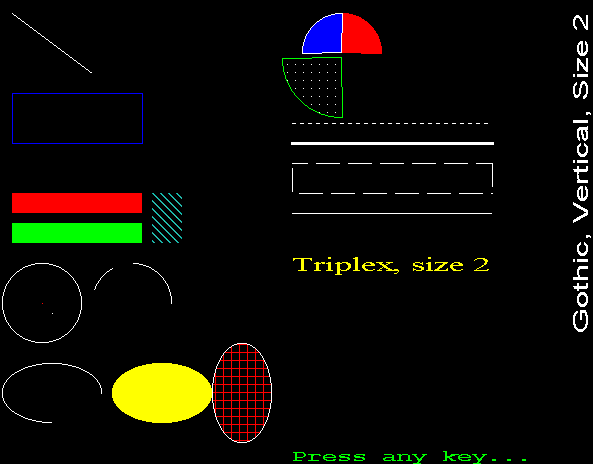